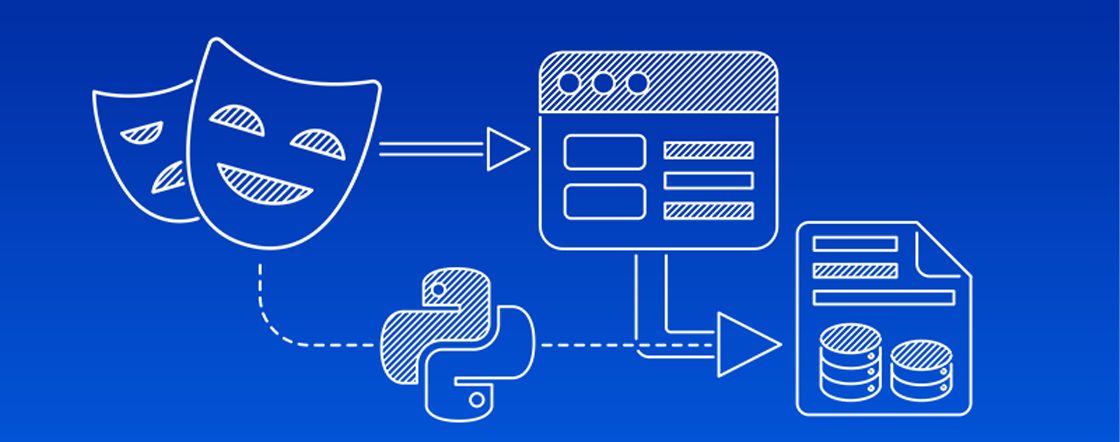
What is End to End Testing?
- End To End Testing is a software testing method that validates entire software from starting to the end along with its integration with external interfaces.
- End To End Testing verifies complete system flow and increases confidence by detecting issues and increasing Test Coverage of subsystems.
- End-to-end testing is an important part of the development process for any web application. It allows you to verify that your application is working correctly from the user’s perspective, by simulating user interactions and verifying that the application behaves as expected.
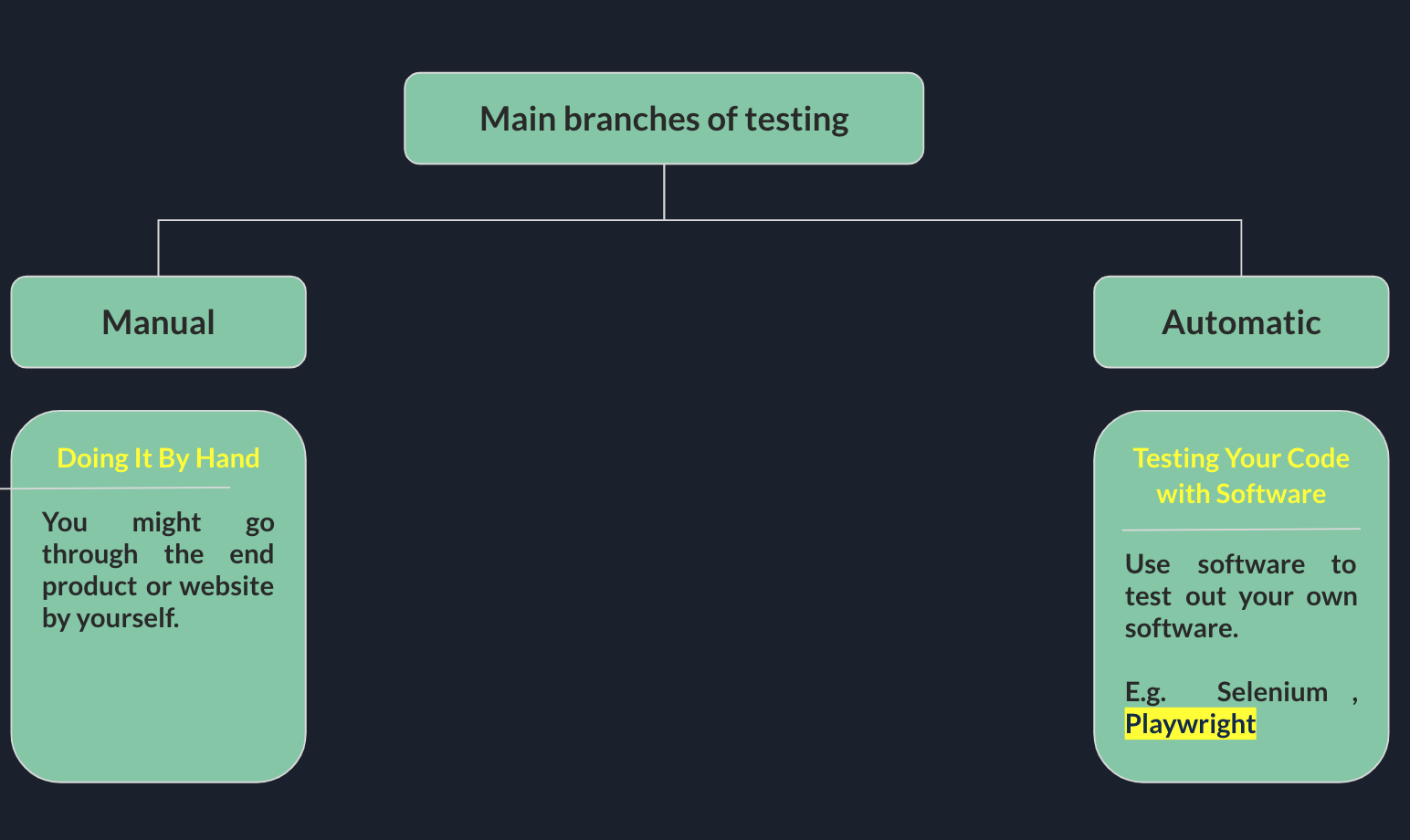
In this blog, we’ll scope discussion on Playwright only. It is a powerful tool for end-to-end testing of web applications, to write test cases for a browser based application. We will take a small example with react by setting up our project and installing the necessary dependencies, and then we’ll write some test cases using Playwright’s API and run them using the command-line interface.
What is Playwright?
- A New Test Automation Framework which is developed by Microsoft for the Modern Web.
- The goal of Playwright Node.js is to provide a single API to developers and testers to automate their web applications across today’s three major browser engines
- Chromium
- Firefox
- WebKit
Feature of Playwright - Any Browser, Any Platform, One API
- Cross-Browser: Playwright supports all modern rendering engines including Chromium, WebKit, and Firefox.
- Cross-Platform: Test on Windows, Linux, and macOS, locally or on CI, headless or headed.
- Cross-Language: Use the Playwright API in TypeScript, JavaScript, Python, .NET, Java.
Powerful Tooling
- Codegen: Generate tests by recording your actions. Save them into any language.
- Playwright Inspector: Inspect page, generate selectors, step through the test execution, see click points, explore execution logs.
- Trace Viewer: Capture all the information to investigate the test failure. Playwright trace contains test execution screencast, live DOM snapshots, action explorer, test source, and many more.
Setting up the Project with React.JS
First, let’s create a new React application or use an existing one. If you don’t have an existing application, you can use the following command to create a new one using,
- Prerequisites
To follow along with this tutorial, you’ll need to have the following software installed on your machine:
- Node.js
- Npm
- Follow below steps to install and configure playwright
1. Create new project using npx create-react-app test-playwright.
npx create-react-app my-app cd my-app
2. Open the project in VSCode and add some basic components in the app.js file like form, textbox and button etc.
3. Open terminal and install below 2 packages for playwright by following command.
npm install @playwright/test npm install playwright
4. Add new “tests” directory in src directory of project (All tests cases are to be placed into this directory).
5. In the test folder, add sample test file by .spec.js and add below code.
const {chromium} = requireC'playwright'); Casync O = { // Launch a new browser instance const browser = await chromium. Launch(); // Create a new page in the browser const page = await browser.newPageQ); // Navigate to the React application await page.gotoC'http://Localhost:3000'); // Click on the button await page.clickC'button'); // Nerify that the message is displayed const message = await page.$eval('#message', Cel) => el.textContent); assert.strictEqual(message, ‘Hello, world!'); // Close the browser await browser.close();
This test case does the following:
a. Launches a new browser instance using Playwright’s chromium.launch() method.
b. Creates a new page in the browser using browser.newPage().
c. Navigates to the React application using page.goto().
d. Clicks on the button using page.click().
e. Verifies that the message is displayed by using page.$eval() to retrieve the text content of the #message element.
Important Terminologies of Playwright
-
Inspector
- Playwright Inspector is a GUI tool that helps authoring and debugging Playwright scripts.
- To run test cases run npx playwright test
- To Open Inspector set environment $env:PWDEBUG=1
-
Trace Generator/Running Codegen
- Playwright comes with the ability to generate tests out of the box.
- npx playwright codegen
- Run codegen and perform actions in the browser. Playwright will generate the code for the user interactions. Codegen will attempt to generate resilient text-based selectors.
-
Trace Viewer
- Playwright Trace Viewer is a GUI tool that helps explore recorded Playwright traces after the script runs.
- If a test fails then it generates a trace file in the result folder.
- To View html report of trace, run npx playwright show-report
Playwright Test Cases for Advanced Configuration using Config File
-
Recording a trace
- ‘off’ – Do not record a trace.
- ‘on’ – Record a trace for each test.
- ‘retain-on-failure’ – Record a trace for each test, but remove it from successful test runs.
- ‘on-first-retry’ – Record a trace only when retrying a test for the first time.
-
Global setup and teardown
- To set something up once before running all tests, use the globalSetup option in the configuration file.
-
Same tests, different configuration
- Same test with different browsers
Refer A Few More Advance Testing Topics with Playwright Here:
- API testing
-
Assertions
- Playwright Test uses expect library for test assertions. This library provides a lot of matchers like toEqual, toContain, toMatch, toMatchSnapshot and many more CDP Session
- CDP Session (Chrome Devtools Protocol)
- ConsoleMessage
- Dialog
- Download and Upload Files
- ElementHandle
- Mouse Events – click,move,down,up etc.
- Keyboard Events
- WebSocket
- Authentication
Playwright vs Selenium
Criteria | Playwright | Selenium |
---|---|---|
Browser Support | Chromium, Firefox, and WebKit | Chrome, Firefox, Opera, Edge, and IE. |
Supported Languages | Playwright supports Java, Python, .Net C#, JavaScript and Typescript | Java, Python, .Net C#, and JavaScript, and it has support for three additional languages, including Ruby, PHP, and Perl. |
Architecture | Playwright has a Headless browser with event-driven architecture. | Selenium has layers architecture based on JSON wire protocol. |
Prerequisites | Need NodeJS to be in fully functional mode. | Selenium requires a standalone selenium server or Client Language Bindings, and browser drivers. |
Speed of Script Execution | Script execution in Playwright is faster compared to Selenium. | Selenium is comparatively slower because the driver serves as a middleman who translates the test script commands and sends them to the browser for execution. |
Conclusion:
Playwright is a powerful tool for creating unit test cases for React applications. Its ability to simulate user interactions and automate browser actions makes it an ideal choice for testing complex user interfaces. The sample code provided in this blog demonstrates how easy it is to set up a playwright and start writing test cases for a React application. By leveraging the capabilities of playwright, developers can ensure that their applications are robust and reliable, and provide a better user experience for their users. Overall, Playwright is a valuable addition to any React developer’s toolkit.
Mr. Radhik Bhojani