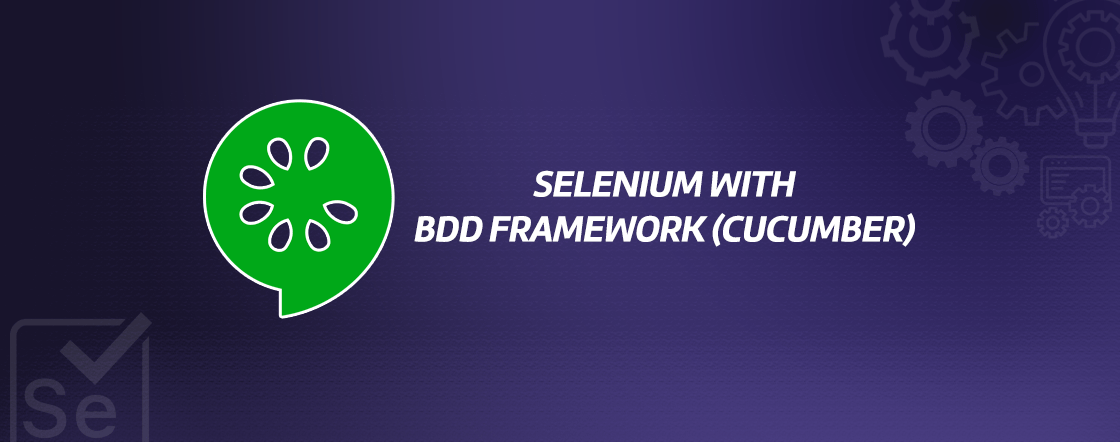
In this dynamic digital landscape, automating tests is crucial for ensuring the reliability and efficiency of software applications. Selenium, a powerful automation tool, combined with the Behavior-Driven Development (BDD) framework, Cucumber, offers a robust approach to testing. In this comprehensive guide, we’ll explore how this tandem simplifies test creation, enhances collaboration between teams, and empowers you to create automated tests that not only find bugs but also document application behavior in plain language. Join us on this journey to streamline your testing processes and elevate software quality.
BDD - Behavior Driven Development
- It is a technique of agile software development in which test cases are written in a simple text language that even non-programmers can understand.
- The aim of a Behavior-Driven Development (BDD) framework is to unite diverse project roles, including quality assurance professionals, developers, and business analysts, in order to gain a comprehensive understanding of the application without delving excessively into its technical complexities.
- Testers create automated test cases using plain language that customers can easily understand.BDD test automation frameworks then interpret these executable specifications written in straightforward English to verify the success of these tests.One of such framework tools is CUCUMBER.
Cucumber – A BDD Framework Tool
- Cucumber is a Behavior Driven Development (BDD) framework tool to write test cases. It works based on Given – When – Then Approach.
- Gherkin is a plain English text language, which helps the tool – Cucumber to interpret and execute the test scripts.
BDD Framework Integration Available with Tools
- Test Framework : TestNG, JUnit
- Build Tools : Maven,Ant
- CI/CD – Jenkins
CUCUMBER ANNOTATIONS: Annotations are predefined text which contain specific meaning. It allows the compiler what should be done during execution.
- Given: Some given context (Preconditions).
- When: Some Action is performed (Actions).
- Then: Output after the above step (Results).
- And : Between any two statements, it gives the logical AND condition. AND can be combined with the GIVEN, WHEN, and THEN statements.
- But : Between any two statements, it gives the logical OR condition.
Steps To Use The Selenium With Cucumber Framework

1. Setup
- JDK
- Eclipse
- Install the cucumber plugin from eclipse Marketplace
2. Create Maven project in eclipse and create package for Java and feature file
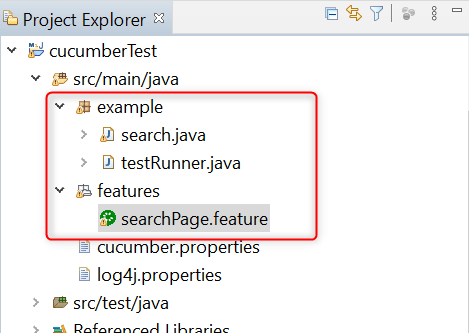
-
Cucumber-java
-
Cucumber-junit / TestNG
4. Create Folder Features & Create feature file in that (Example : searchFeature.features) which contains scenario as per given Condition (Given, When, Then)
SearchPage. feature Feature: Test Google Search Functionality Scenario: Search and verify page title Given #. User is on Google Search page When #. User search with cucumber framwork keyword And #. Click on selection options Then #. User is redirected to search result page
package example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; import org.testng.annotations.Test; import io.cucumber.java.After; import io.cucumber.java.Before; import io.cucumber.java.en.And; import io.cucumber.java.en.Given; import io.cucumber.java.en.Then; import io.cucumber.java.en.When; import io.github.bonigarcia.wdm.WebDriverManager; public class search { private static WebDriver driver; @Before public void setUp() throws InterruptedException { WebDriverManager.chromedriver().setup(); driver = new ChromeDriver(); Thread.sleep(10); driver.manage().window().maximize(); } @Test public void checkTest() { System.out.println("Testing"); } @Given("#. User is on Google Search page") public void open_login_page() throws Throwable { driver.get("https://www.google.co.in/"); System.out.println("1. Go to Google search page"); } @When("#. User search with cucumber framwork keyword") public void enter_credentails() throws Throwable { System.out.println("2. Search with cucumber framwork keyword."); driver.findElement(By.xpath(".//textarea[@title='Search']")).sendKeys("cucumber framework"); Thread.sleep(10); driver.findElement(By.xpath(".//img[@alt='Google']")).click(); Thread.sleep(10); } @And("#. Click on selection options") public void click_login(){ System.out.println("3. Click on Google search button"); driver.findElement(By.xpath("(.//input[@value='Google Search'])[last()]")).click(); } @Then("#. User is redirected to search result page") public void redirect_homePage() throws Throwable { System.out.println("4. User is redirected to search result page"); String actTitle = driver.getTitle(); String expTitle = "cucumber framework - Google Search"; Assert.assertEquals(actTitle,expTitle); } /* * @After public void teardown() { driver.quit(); } */ }
6. Run feature file with Run as cucumber feature option to execute the scenario
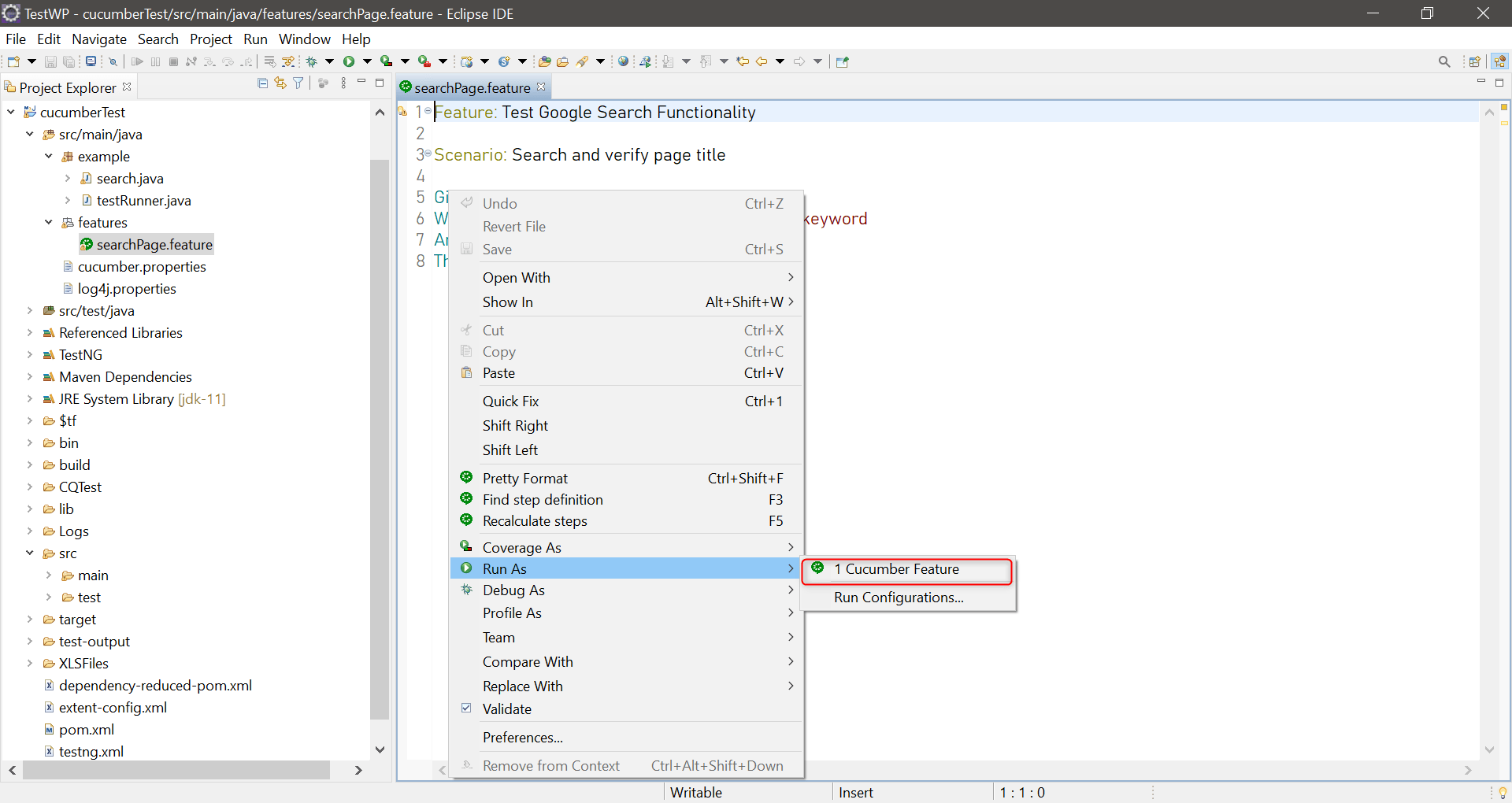
Annotations used- Cucumber Options
- Features – Path of feature file
- Glue- path of Java class
File Name : testRunner.java package example; import io.cucumber.testng.AbstractTestNGCucumberTests; import io.cucumber.testng.CucumberOptions; @CucumberOptions(features="src/main/java/features/searchPage.feature", glue={"example"}, plugin = { "pretty", "html:target/cucumber-reports" }, monochrome = true) public class testRunner extends AbstractTestNGCucumberTests{ }
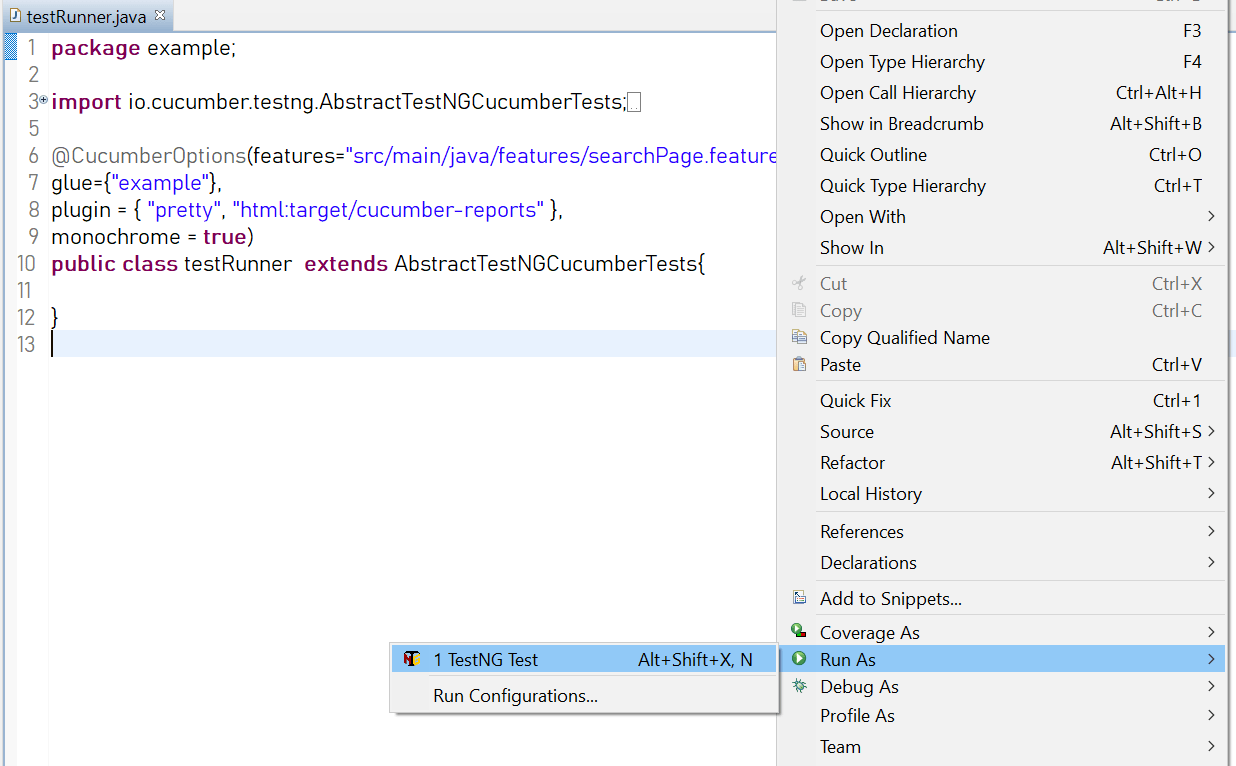
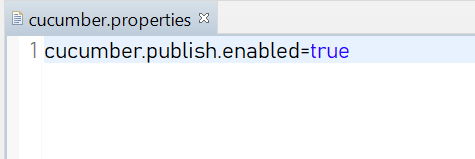
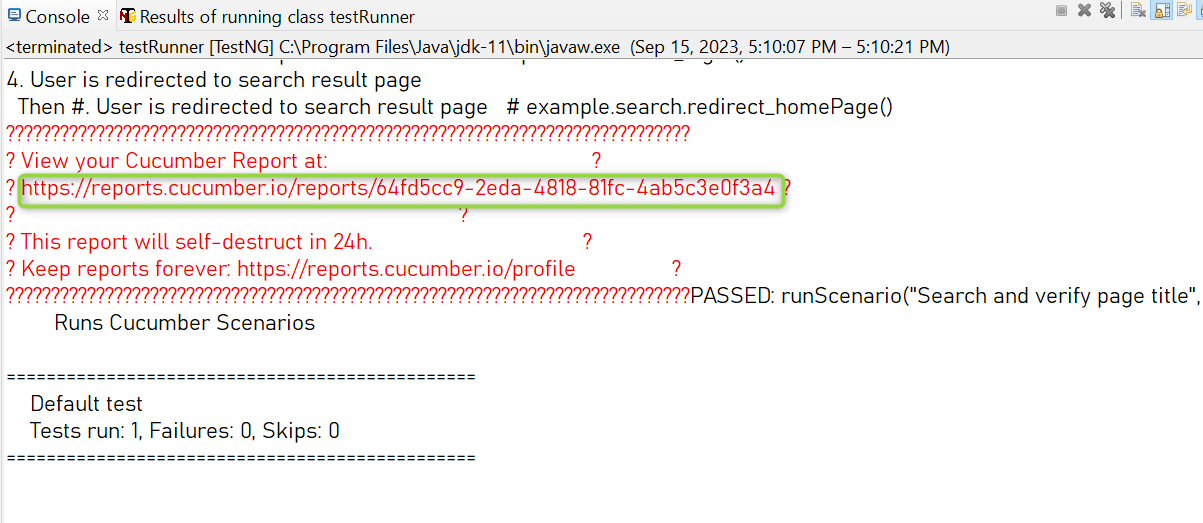
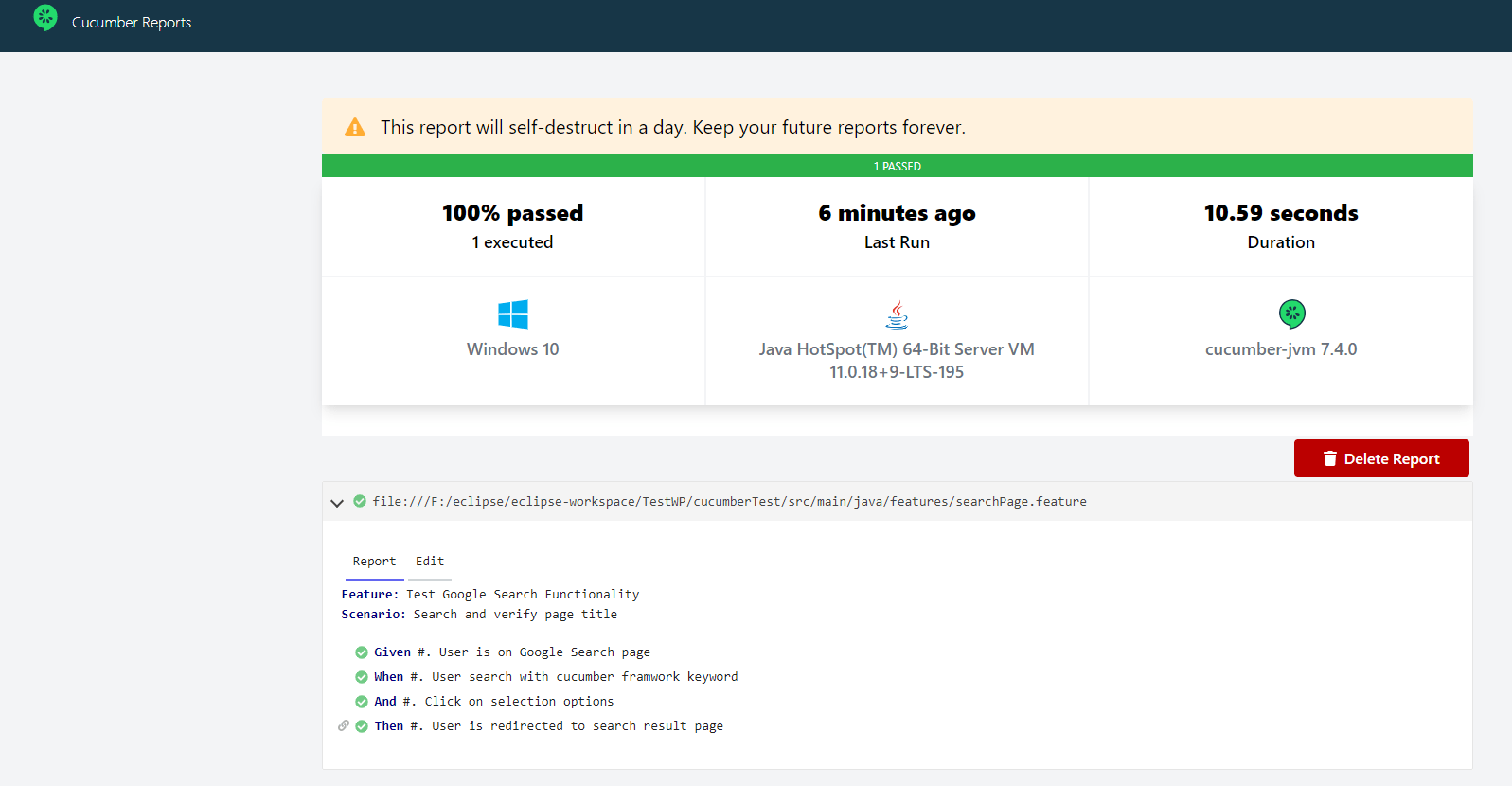
Payal Nanavati